Enable back in stock for custom catalog feeds
Learn how to enable back in stock tracking for custom catalog feed items based on inventory quantity, so you can send email alerts to customers interested in purchasing out of stock items.
Before getting started
If you’ve already set up a custom catalog feed in Klaviyo, continue with the steps below to enable back in stock. Note that some of the steps needed to enable back in stock are in the configuration stage; they are outlined under Optional fields in the custom catalog setup guide, and reiterated below in this article.
Feed setup
When you set up your custom catalog feed, make sure to include the following field for each item in your feed:
- Inventory (
$inventory_quantity
): a number representing the stock available for a given item.
You can also include the following optional parameter:
- Inventory Policy (
$inventory_policy
): a number that controls how we treat product visibility in product feeds/blocks. This field has two options:- If the policy is set to 1, a product will not appear in dynamic product recommendation feeds and blocks if it is out of stock.
- If the policy is set to 2, a product can appear in dynamic product recommendation feeds and blocks regardless of inventory quantity. This is also the default behavior when this field is not set.
During the field mapping step, these fields should have the feed item fields: inventory_quantity and inventory_policy mapped to the Klaviyo fields $inventory_quantity
and $inventory_policy
respectively, and the field type should be Number.

Account settings and flow triggering
Once you've setup your feed and a Subscribed to Back in Stock event has been captured, the Back in Stock Settings will appear under Account > Settings > Other in your account. If you don't see these settings, make sure to trigger an event first. You can use these settings to determine how you would like to trigger back in stock flows.
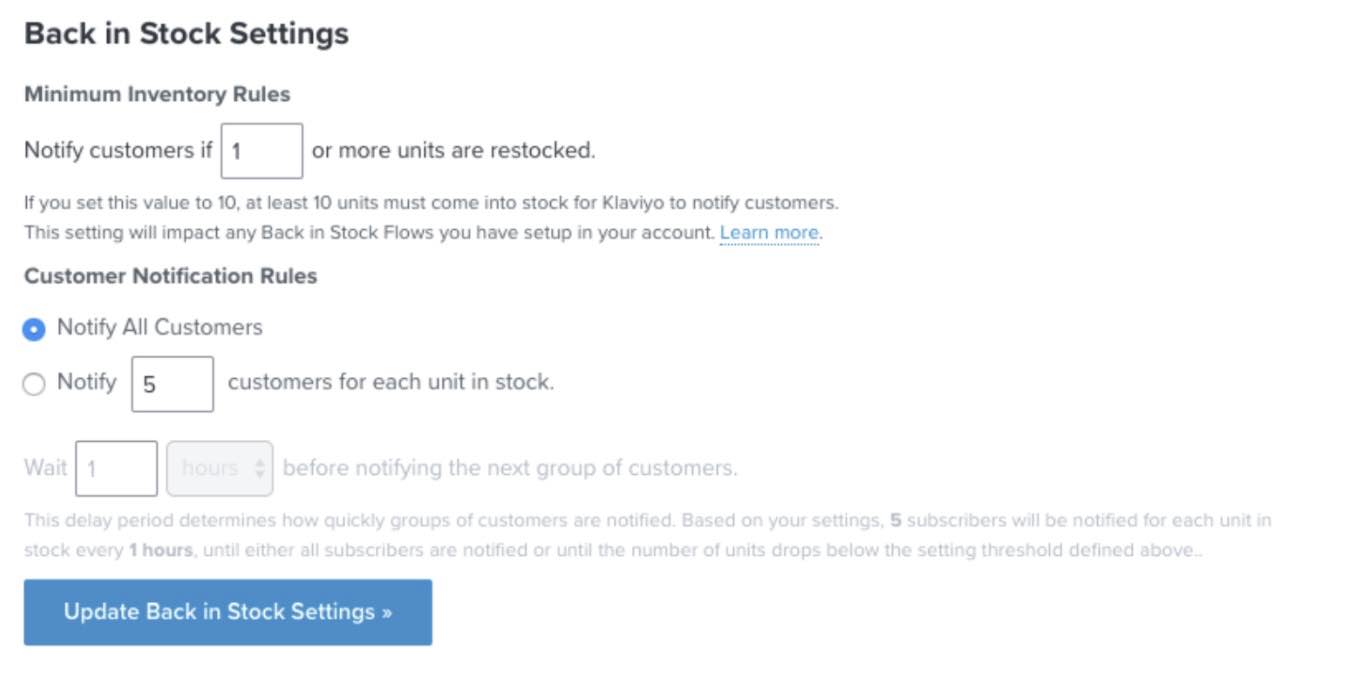
To set up a back in stock alert flow, take a look at our How to build a back in stock flow guide.
Subscribing customers to back in stock alerts
When a customer subscribes to an alert when an item returns in stock, send a request to our back in stock endpoint. Learn how to set up server-side and client-side requests with our guide on how to set up back in stock for custom catalog feeds. For more information on which you should use, check out our custom integration FAQ.
Server-side request
To send subscription information to the server-side API, use a Create Back In Stock Subscription request. The JSON request payload should look like the example below and contain the following:
channels
- an array containing the channels through which the profile would like to receive back in stock notifications (email, SMS, and/or push).profile
- the profile of the person subscribing to alerts for the item (containing at least one profile identifier(s) for back in stock notifications).relationships
- the object containing the catalog variant related to the back in stock subscription.
{
"data": {
"type": "back-in-stock-subscription",
"attributes": {
"channels": [
"SMS"
],
"profile": {
"data": {
"type": "profile",
"attributes": {
"email": "sarah.mason@klaviyo-demo.com"
},
"id": "01GDDKASAP8TKDDA2GRZDSVP4H"
}
}
},
"relationships": {
"variant": {
"data": {
"type": "catalog-variant",
"id": "$custom:::$default:::SAMPLE-DATA-ITEM-1-VARIANT-MEDIUM"
}
}
}
}
}
Client-side request
To send subscription information to the client-side API, use a Create Client Back In Stock Subscription request. The JSON request payload should look like the example below and contain the following:
channels
- an array containing the channels through which the profile would like to receive back in stock notifications (email, SMS, and/or push).profile
- the profile of the person subscribing to alerts for the item (containing at least one profile identifier(s) for back in stock notifications).relationships
- the object containing the catalog variant related to the back in stock subscription.
const fetch = require('node-fetch');
const url = 'https://a.klaviyo.com/client/back-in-stock-subscriptions/?company_id=PUBLIC_API_KEY';
const options = {
method: 'POST',
headers: {
accept: 'application/json',
revision: '2024-02-15',
'content-type': 'application/json'
},
body: JSON.stringify({
data: {
type: 'back-in-stock-subscription',
attributes: {
channels: ['EMAIL'],
profile: {
data: {
type: 'profile',
id: '01GDDKASAP8TKDDA2GRZDSVP4H',
attributes: {
email: 'sarah.mason@klaviyo-demo.com',
phone_number: '+15005550006',
external_id: '63f64a2b-c6bf-40c7-b81f-bed08162edbe'
}
}
}
},
relationships: {
variant: {
data: {
type: 'catalog-variant',
id: '$custom:::$default:::SAMPLE-DATA-ITEM-1-VARIANT-MEDIUM'
}
}
}
}
})
};
fetch(url, options)
.then(res => res.json())
.then(json => console.log(json))
.catch(err => console.error('error:' + err));
Invalid variants & duplicate subscriptions
- If an invalid variant ID for an item is included in a request, the API will return an error response. No event will be created for the profile, and the customer will not be queued to receive a back in stock message.
- It is not possible to submit multiple subscriptions for a profile on the same item variant. If multiple requests are submitted for the same email address on the same variant, only the first will be accepted.
- There is no limit to the number of unique item variants a profile can subscribe to.
- After an individual receives an email notifying them that a specific variant is back in stock, their existing subscription is cleared. They are then able to sign up for another subscription on that same variant.
Additional resources
Updated 7 months ago