Make a test API request
Test your connection to confirm your authentication is working and that your application can connect with Klaviyo's servers.
Test POST request
To check that your integration is working correctly, make a test request to the List API using the code below to create a new list. Replace the PRIVATE_KEY
value with your ow private API key. If you are using a sandbox account, first check the key is associated with your sandbox account. Assign a test name to the list_name
form data, for example Test List
.
A successful request returns the list ID of the newly created list.
curl --request POST \
--url 'https://a.klaviyo.com/api/v2/lists?api_key=PRIVATE_KEY' \
--header 'Accept: application/json' \
--header 'Content-Type: application/x-www-form-urlencoded' \
--data list_name=MyNewList
import requests
url = "https://a.klaviyo.com/api/v2/lists"
querystring = {"api_key":"PRIVATE_KEY"}
payload = "list_name=MyNewList"
headers = {
"Accept": "application/json",
"Content-Type": "application/x-www-form-urlencoded"
}
response = requests.request("POST", url, data=payload, headers=headers, params=querystring)
print(response.text)
<?php
$curl = curl_init();
curl_setopt_array($curl, [
CURLOPT_URL => "https://a.klaviyo.com/api/v2/lists?api_key=PRIVATE_KEY",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => "",
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 30,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => "POST",
CURLOPT_POSTFIELDS => "list_name=MyNewList",
CURLOPT_HTTPHEADER => [
"Accept: application/json",
"Content-Type: application/x-www-form-urlencoded"
],
]);
$response = curl_exec($curl);
$err = curl_error($curl);
curl_close($curl);
if ($err) {
echo "cURL Error #:" . $err;
} else {
echo $response;
}
require 'uri'
require 'net/http'
require 'openssl'
url = URI("https://a.klaviyo.com/api/v2/lists?api_key=PRIVATE_KEY")
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
request = Net::HTTP::Post.new(url)
request["Accept"] = 'application/json'
request["Content-Type"] = 'application/x-www-form-urlencoded'
request.body = "list_name=MyNewList"
response = http.request(request)
puts response.read_body
{
"list_id": "LIST_ID"
}
Test with Postman
You can find a collection of prebuilt API requests in the Klaviyo Postman workspace. Export and import into your local Postman client, or fork the Klaviyo API collection into your personal workspace to get started.
To confirm that your application can send data to Klaviyo, open the Lists & Segments folder and select the Create List POST request. Replace the PRIVATE_KEY
value with a private API key from your account. If you are using a test account, first check the key is associated with your test account. In the body data, replace the MyNewList
value with your preferred test list name, for example Test List
.
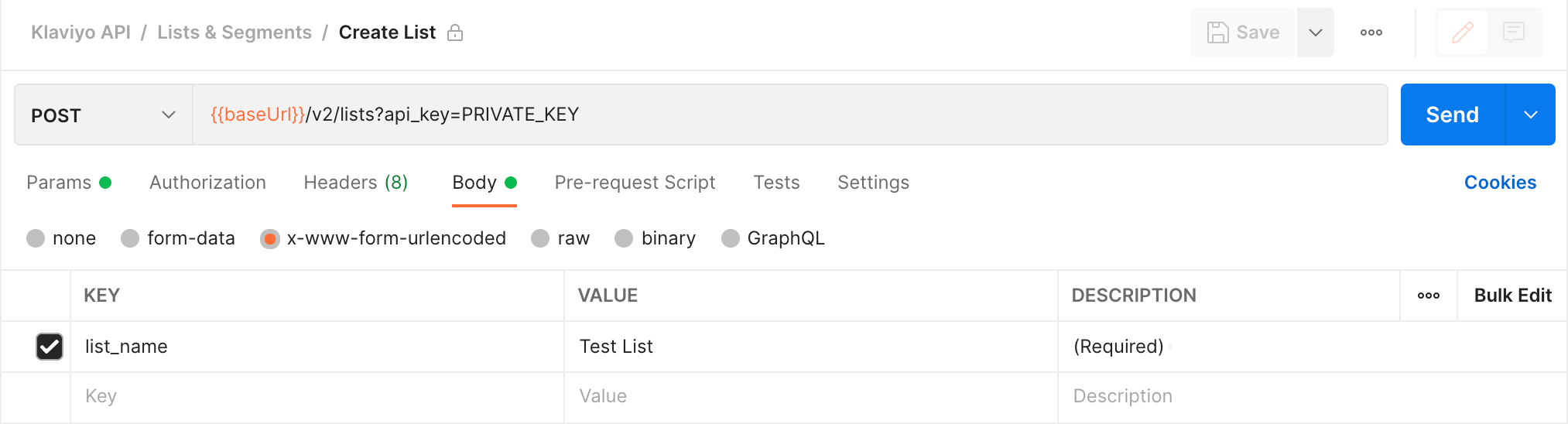
{
"list_id": "LIST_ID"
}
Confirm success in account
Double-check that your test request successfully created a list in your preferred account. While logged in to your account, navigate to Lists & Segments a search for the name of your newly-created Test List
to verify.
Updated 6 months ago